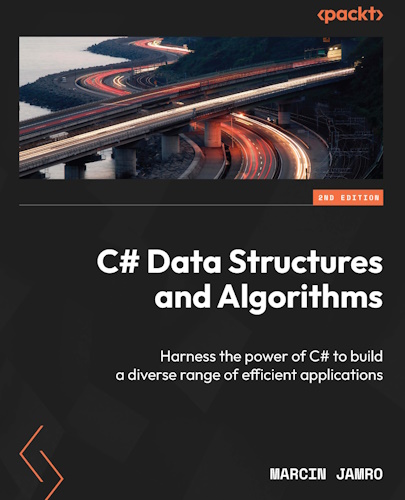
This book is for developers looking to learn data structures and algorithms in C#. While basic programming skills and C# knowledge is useful, beginners will find value in the provided code snippets, illustrations, and detailed explanations, enhancing their programming skills. Advanced developers can use this book as a valuable resource for reusable code snippets, instead of writing algorithms from scratch each time.
Write complex code in C# with this complete guide to using various data structures and algorithms, containing ready-to-use code snippets, detailed explanations, and illustrations
Key features
Basic lists, stacks, queues, dictionaries, sets, and trees, among other data structures
Learn efficient design and implementation techniques to suit your software requirements
Visualize data structures and algorithms with illustrations to better understand their analysis
Book Description
Building your own applications is fun but also challenging, especially when solving complex problems involving advanced data structures and algorithms. This endeavor requires deep knowledge of the programming language, as well as data structures and algorithms, which is exactly what this book offers C# developers.
Starting with an introduction to algorithms, this book gradually introduces you to the world of arrays, lists, stacks, queues, dictionaries, and sets. Real-life examples, complemented by code snippets and illustrations, provide practical understanding of these concepts. You will also learn how to sort arrays using various algorithms, which will lay a solid foundation for your programming knowledge. As you progress through the book, you'll become familiar with more complex data structures—trees and graphs—and discover algorithms for problems such as finding the shortest path in a graph, before moving on to see different algorithms in action, such as solving Sudoku.
By the end of the book, you'll learn how to use the C# language to create algorithmic components that are not only easy to understand and debug, but also easily applicable to a variety of applications spanning web and mobile platforms.
What you'll learn
Understand the basics of algorithms and their classification
Store data using arrays and lists, and learn different ways to sort arrays
Create advanced applications with stacks, queues, hash tables, dictionaries, and sets
Create efficient applications with tree-based algorithms, such as search binary search tree
Increase the efficiency of solving using graphs, including finding the shortest path on a graph
Implement algorithms that solve problems in the Tower of Hanoi and Sudoku games, generate fractals and even guess the title of this book
Who is this book for?
This book is intended for developers who want to learn data structures and algorithms in C#. While basic programming skills and knowledge of C# are helpful, beginners will find value in the code snippets, illustrations, and detailed explanations provided to enhance their programming skills. Advanced developers can use this book as a valuable resource for reusing pieces of code rather than having to write algorithms from scratch each time.
You must reply in thread to view hidden text or upgrade your account to always see hidden content.